How to automatically send email based on cell value in Excel?
Supposing you want to send an email through Outlook to a certain recipient based on a specified cell value in Excel. For example, when the value of cell D7 in a worksheet is greater than 200, then an email is created automatically. This article introduces a VBA method for you to quickly solve this issue.
Automatically send email based on cell value with VBA code
Automatically send email based on cell value with VBA code
Please do as follows to send an email based on cell value in Excel.
1. In the worksheet you need to send email based on its cell value (here says the cell D7), right-click the sheet tab, and select View Code from the context menu. See screenshot:
2. In the popping up Microsoft Visual Basic for Applications window, please copy and paste the below VBA code into the sheet code window.
VBA code: Send email through Outlook based on cell value in Excel
Dim xRg As Range
'Update by Extendoffice 2018/3/7
Private Sub Worksheet_Change(ByVal Target As Range)
On Error Resume Next
If Target.Cells.Count > 1 Then Exit Sub
Set xRg = Intersect(Range("D7"), Target)
If xRg Is Nothing Then Exit Sub
If IsNumeric(Target.Value) And Target.Value > 200 Then
Call Mail_small_Text_Outlook
End If
End Sub
Sub Mail_small_Text_Outlook()
Dim xOutApp As Object
Dim xOutMail As Object
Dim xMailBody As String
Set xOutApp = CreateObject("Outlook.Application")
Set xOutMail = xOutApp.CreateItem(0)
xMailBody = "Hi there" & vbNewLine & vbNewLine & _
"This is line 1" & vbNewLine & _
"This is line 2"
On Error Resume Next
With xOutMail
.To = "Email Address"
.CC = ""
.BCC = ""
.Subject = "send by cell value test"
.Body = xMailBody
.Display 'or use .Send
End With
On Error GoTo 0
Set xOutMail = Nothing
Set xOutApp = Nothing
End Sub
Notes:
3. Press the Alt + Q keys together to close the Microsoft Visual Basic for Applications window.
From now on, when the value you entering in cell D7 is greater than 200, an email with specified recipients and body will be created automatically in Outlook. You can click the Send button to send this email. See screenshot:
Notes:
1. The VBA code is only working when you use Outlook as your email program.
2. If the entered data in cell D7 is a text value, the email window will be popped out as well.
Easily send email through Outlook based on fields of created mailing list in Excel:
The Send Emails utility of Kutools for Excel helps users sending email through Outlook based on created mailing list in Excel.
Download and try it now! (30-day free trail)
Related articles:
- How to send an email through Outlook when workbook is saved in Excel?
- How to send email if a certain cell is modified in Excel?
- How to send email if button is clicked in Excel?
- How to send email if due date has been met in Excel?
- How to send email reminder or notification if workbook is updated in Excel?
Best Office Productivity Tools
Supercharge Your Excel Skills with Kutools for Excel, and Experience Efficiency Like Never Before. Kutools for Excel Offers Over 300 Advanced Features to Boost Productivity and Save Time. Click Here to Get The Feature You Need The Most...
Office Tab Brings Tabbed interface to Office, and Make Your Work Much Easier
- Enable tabbed editing and reading in Word, Excel, PowerPoint, Publisher, Access, Visio and Project.
- Open and create multiple documents in new tabs of the same window, rather than in new windows.
- Increases your productivity by 50%, and reduces hundreds of mouse clicks for you every day!

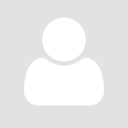
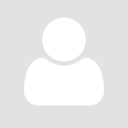
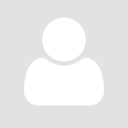
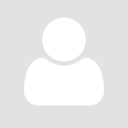
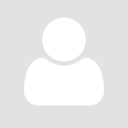
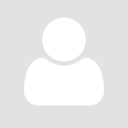
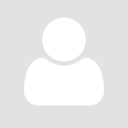
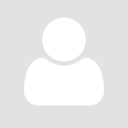